Overview
coincheck allows roughly two kinds of APIs; Public API and Private API.
Public API allows you to browse order status and order book.
Private API allows you to create and cancel new orders, and to check your balance.
Request URL
https://coincheck.com
Authentication
Please authenticate to use a Private API.
Generate API Key
First, please create your API Key. You can create one here.
Please don't share your Access key and Secret Access key with others
Make a request using these keys.
Permission settings
By generating an API key, you can set a permission for each function.
And you can also give permission to IP addresses you prefer.
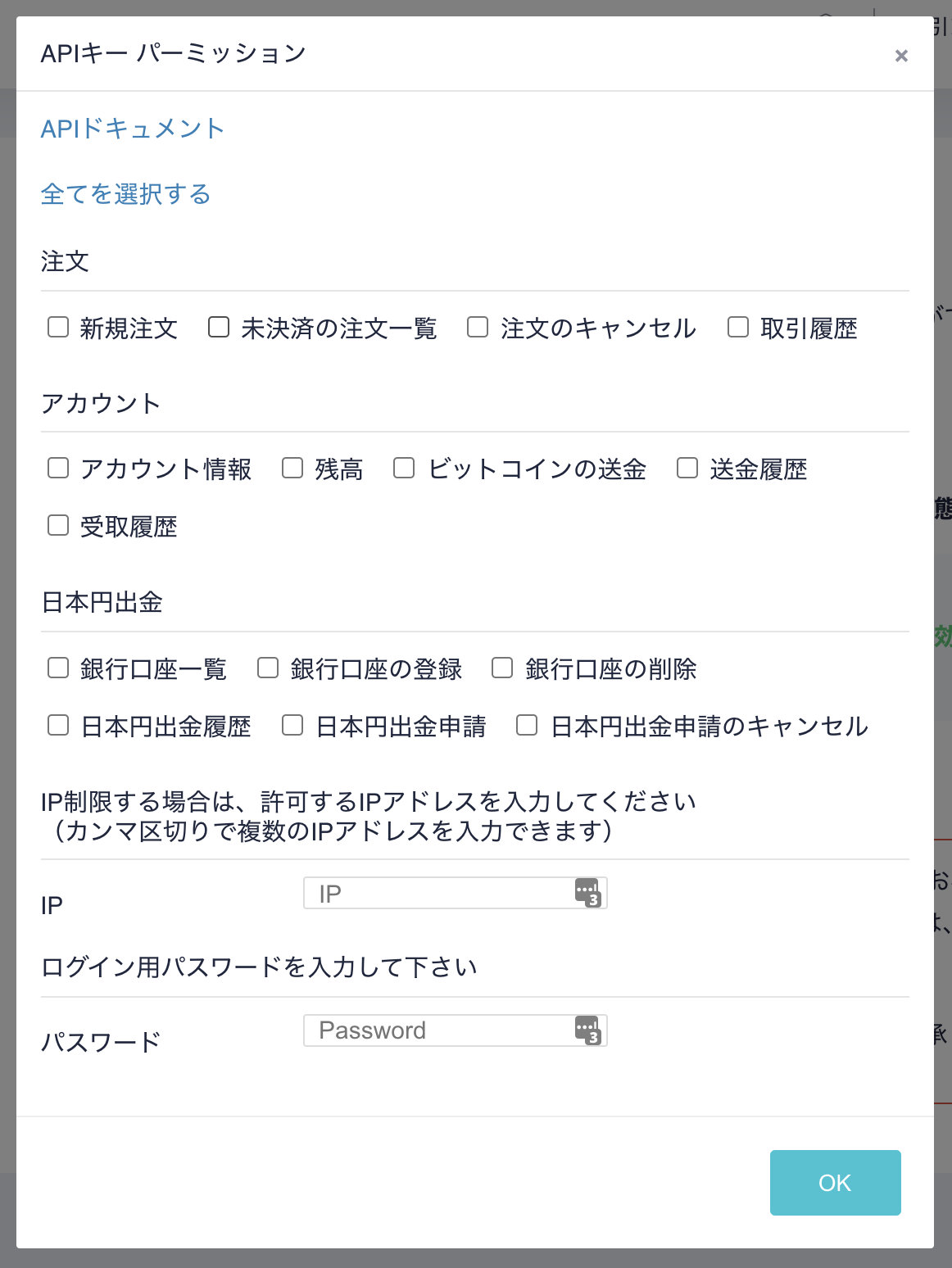
Making a Request
For requests that require authentication, you have to add information below to HTTP Header.
- ACCESS-KEY Access key you genertaed at API key
- ACCESS-NONCE Positive integer will increase every time you send request (managed for each API key). A common practice is to use UNIX time. Maximum value is 9223372036854775807.
- ACCESS-SIGNATURE SIGNATURE mentioned later
Create Signature
SIGNATURE is a HMAC-SHA256 encoded message containing: ACCESS-NONCE, Request URL and Request body by using API key.
Ruby
require "openssl" nonce = Time.now.to_i.to_s url = "https://coincheck.com/api/accounts/balance" body = "hoge=foo" message = nonce + url + body secret = "API_SECRET" OpenSSL::HMAC.hexdigest(OpenSSL::Digest.new("sha256"), secret, message) # => "3919705fea4b0cd073b9c6e01e139f3b056782c57c5cffd5aea6d8c4ac98bef7"
PHP
$strUrl = "https://coincheck.com/api/accounts/balance"; $intNonce = time(); $arrQuery = array("hoge" => "foo"); $strAccessSecret = "API_SECRET"; $strMessage = $intNonce . $strUrl . http_build_query($arrQuery); $strSignature = hash_hmac("sha256", $strMessage, $strAccessSecret); # => "3bc1f33d802056c61ba8c8108f6ffb7527bcd184461a3ea0fed3cee0a22ae15d"
Request sample
Ruby
require 'net/http' require 'uri' require 'openssl' key = "API_KEY" secret = "API_SECRET" uri = URI.parse "https://coincheck.com/api/accounts/balance" nonce = Time.now.to_i.to_s message = nonce + uri.to_s signature = OpenSSL::HMAC.hexdigest(OpenSSL::Digest.new("sha256"), secret, message) headers = { "ACCESS-KEY" => key, "ACCESS-NONCE" => nonce, "ACCESS-SIGNATURE" => signature } https = Net::HTTP.new(uri.host, uri.port) https.use_ssl = true response = https.start { https.get(uri.request_uri, headers) } puts response.body
Java
import com.google.api.client.http.*; import com.google.api.client.http.apache.ApacheHttpTransport; import com.google.api.client.json.jackson2.JacksonFactory; import org.apache.commons.codec.binary.Hex; import javax.crypto.Mac; import javax.crypto.spec.SecretKeySpec; import java.io.IOException; import java.security.InvalidKeyException; import java.security.NoSuchAlgorithmException; import java.util.HashMap; import java.util.Map; public class CoincheckApi { private String apiKey; private String apiSecret; public static void main(String[] args) { String key = "API_KEY"; String secret = "API_SECRET"; CoincheckApi api = new CoincheckApi(key, secret); System.out.println(api.getTicker()); System.out.println(api.getBalance()); } public CoincheckApi(String apiKey, String apiSecret) { this.apiKey = apiKey; this.apiSecret = apiSecret; } public String getTicker() { String url = "https://coincheck.com/api/accounts/ticker"; String jsonString = requestByUrlWithHeader(url, createHeader(url)); return jsonString; } public String getBalance() { String url = "https://coincheck.com/api/accounts/balance"; String jsonString = requestByUrlWithHeader(url, createHeader(url)); return jsonString; } private Map<String, String> createHeader(String url) { Map<String, String> map = new HashMap<String, String>(); String nonce = createNonce(); map.put("ACCESS-KEY", apiKey); map.put("ACCESS-NONCE", nonce); map.put("ACCESS-SIGNATURE", createSignature(apiSecret, url, nonce)); return map; } private String createSignature(String apiSecret, String url, String nonce) { String message = nonce + url; return HMAC_SHA256Encode(apiSecret, message); } private String createNonce() { long currentUnixTime = System.currentTimeMillis() / 1000L; String nonce = String.valueOf(currentUnixTime); return nonce; } private String requestByUrlWithHeader(String url, final Map<String, String> headers){ ApacheHttpTransport transport = new ApacheHttpTransport(); HttpRequestFactory factory = transport.createRequestFactory(new HttpRequestInitializer() { public void initialize(final HttpRequest request) throws IOException { request.setConnectTimeout(0); request.setReadTimeout(0); request.setParser(new JacksonFactory().createJsonObjectParser()); final HttpHeaders httpHeaders = new HttpHeaders(); for (Map.Entry<String, String> e : headers.entrySet()) { httpHeaders.set(e.getKey(), e.getValue()); } request.setHeaders(httpHeaders); } }); String jsonString; try { HttpRequest request = factory.buildGetRequest(new GenericUrl(url)); HttpResponse response = request.execute(); jsonString = response.parseAsString(); } catch (IOException e) { e.printStackTrace(); jsonString = null; } return jsonString; } public static String HMAC_SHA256Encode(String secretKey, String message) { SecretKeySpec keySpec = new SecretKeySpec( secretKey.getBytes(), "hmacSHA256"); Mac mac = null; try { mac = Mac.getInstance("hmacSHA256"); mac.init(keySpec); } catch (NoSuchAlgorithmException e) { // can't recover throw new RuntimeException(e); } catch (InvalidKeyException e) { // can't recover throw new RuntimeException(e); } byte[] rawHmac = mac.doFinal(message.getBytes()); return Hex.encodeHexString(rawHmac); } }
Libraries
We developed a library for each programming language to be helpful when You use API of coincheck and distribute it.
Ruby
Librariy is available, if you execute the following commands than a command-line or
$ gem install ruby_coincheck_client
if you describe it as follows in Gemfile under Bundler environment.
gem 'ruby_coincheck_client'
We can download the gem file from GitHub.
coincheckjp/ruby_coincheck_client
PHP
Add the following to composer.json.
{ "require": { "coincheck/coincheck": "1.0.0" } }
Package install
$ composer install
We can download the package file from GitHub.
Scala
val key = "YOUR-KEY-HERE" val secret = "YOUR-SECRET-HERE" import net.pocketengineer.coincheckscala.CoinCheckApiService import net.pocketengineer.coincheckscala.model._ val cc = new CoinCheckApiService(key, secret) // Ticker val ticker: Ticker = cc.getTicker // OrderBook val orderBook: OrderBook = cc.getOrderBook // Account Info val balance: Balance = cc.getAccountInfo // Trade val orderId: Long = cc.tradeBtc(28400, 0.01F, BUY) // OpenOrder val openOrders: List[OpenOrder] = cc.getOpenOrder // Cancel Order val isSuccess: Boolean = cc.cancelOrder(ORDER_ID)
Build
sbt assembly
Pagination
At Some API pagination of coincheck It is possible to get by dividing the data.
PARAMETERS
- limit You can specify the number of acquisition per page.
- order You can specify "desc" or "asc".
- starting_after If you specify the ID you can set the start point of list.
- ending_before If you specify the ID you can set the start point of list.
{"success":true,"pagination":{"limit":1,"order":"desc","starting_after":null,"ending_before":null},"data":[{"id":10,"pair":"btc_jpy","status":"open","created_at":"2015-12-02T05:27:53.000Z","closed_at":null,"open_rate":"43553.0","closed_rate":null,"amount":"1.51347797","all_amount":"1.51045705","side":"sell","pl":"-8490.81029287","new_order":{"id":23104033,"side":"sell","rate":null,"amount":null,"pending_amount":"0","status":"complete","created_at":"2015-12-02T05:27:52.000Z"},"close_orders":[{"id":23755132,"side":"buy","rate":"10000.0","amount":"1.0","pending_amount":"0.0","status":"cancel","created_at":"2015-12-05T05:03:56.000Z"}]}]}
Public API
Public API allows you to browse order status, order transactions and order book.
Ticker
Check latest information
If pair is not specified, you can get the information of btc_jpy.
HTTP REQUEST
GET /api/ticker
PARAMETERS
- pair Specify a currency pair to trade. btc_jpy, eth_jpy, etc_jpy, lsk_jpy, xrp_jpy, xem_jpy, bch_jpy, mona_jpy, iost_jpy, enj_jpy, chz_jpy, imx_jpy, shib_jpy, avax_jpy, plt_jpy, fnct_jpy, dai_jpy, wbtc_jpy, bril_jpy, bc_jpy, doge_jpy are now available.
{"last":27390,"bid":26900,"ask":27390,"high":27659,"low":26400,"volume":"50.29627103","timestamp":1423377841}
RESPONSE ITEMS
- last Latest quote
- bid Current highest buying order
- ask Current lowest selling order
- high The highest price within 24 hours
- low The lowest price within 24 hours
- volume 24 hours trading volume
- timestamp Current time
Public trades
You can get current order transactions.
HTTP REQUEST
GET /api/trades
PARAMETERS
- *pair Specify a currency pair to trade. btc_jpy, eth_jpy, etc_jpy, lsk_jpy, xrp_jpy, xem_jpy, bch_jpy, mona_jpy, iost_jpy, enj_jpy, chz_jpy, imx_jpy, shib_jpy, avax_jpy, plt_jpy, fnct_jpy, dai_jpy, wbtc_jpy, bril_jpy, bc_jpy, doge_jpy are now available.
{"success":true,"pagination":{"limit":1,"order":"desc","starting_after":null,"ending_before":null},"data":[{"id":82,"amount":"0.28391","rate":"35400.0","pair":"btc_jpy","order_type":"sell","created_at":"2015-01-10T05:55:38.000Z"},{"id":81,"amount":"0.1","rate":"36120.0","pair":"btc_jpy","order_type":"buy","created_at":"2015-01-09T15:25:13.000Z"}]}
Order Book
Fetch order book information.
HTTP REQUEST
GET /api/order_books
{"asks":[[27330,"2.25"],[27340,"0.45"]],"bids":[[27240,"1.1543"],[26800,"1.2226"]]}
RESPONSE ITEMS
- asks Sell order status
- bids Buy order status
Calc Rate
To calculate the rate from the order of the exchange.
HTTP REQUEST
GET /api/exchange/orders/rate
PARAMETERS
- *order_type Order type("sell" or "buy")
- *pair Specify a currency pair to trade. btc_jpy, eth_jpy, etc_jpy, lsk_jpy, xrp_jpy, xem_jpy, bch_jpy, mona_jpy, iost_jpy, enj_jpy, chz_jpy, imx_jpy, shib_jpy, avax_jpy, plt_jpy, fnct_jpy, dai_jpy, wbtc_jpy, bril_jpy, bc_jpy, doge_jpy are now available.
- amount Order amount (ex) 0.1
- price Order price (ex) 28000
- ※ Either price or amount must be specified as a parameter.
{"success":true,"rate": 60000, "price": 60000, "amount": 1.0}
RESPONSE ITEMS
- rate Order rate
- price Order price
- amount Order amount
Standard Rate
Standard Rate of Coin.
HTTP REQUEST
GET /api/rate/[pair]
PARAMETERS
- *pair pair (e.g. "btc_jpy" )
{"rate": "60000"}
RESPONSE ITEMS
- rate rate
Status Retrieval
Retrieving the status of the exchange.
For a description of the exchange status, please refer to this page.
HTTP REQUEST
GET /api/exchange_status
PARAMETERS
- If pair is not specified, information on all tradable pairs is returned.
-
If pair is specified, information is returned only for that particular currency.
- Currently available pairs are btc_jpy, eth_jpy, etc_jpy, lsk_jpy, xrp_jpy, xem_jpy, bch_jpy, mona_jpy, iost_jpy, enj_jpy, chz_jpy, imx_jpy, shib_jpy, avax_jpy, plt_jpy, fnct_jpy, dai_jpy, wbtc_jpy, bril_jpy, bc_jpy, doge_jpy.
{ "exchange_status": [ { "pair": "btc_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "eth_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "etc_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "lsk_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "xrp_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "xem_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "bch_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "mona_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "iost_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "enj_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "chz_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "imx_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "shib_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "avax_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "plt_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "fnct_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "dai_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "wbtc_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "bril_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "bc_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } }, { "pair": "doge_jpy", "status": "available", "timestamp": 1745594573, "availability": { "order": true, "market_order": true, "cancel": true } } ] }
RESPONSE ITEMS
- pair Symbol
- status Exchange status ( available, itayose, stop ).
- timestamp Time of Status Retrieval
- availability Response whether limit orders ( order ), market orders ( market_order ), or cancel orders ( cancel ) can be placed.
Private API
Private API allows you to order, cancel new orders and make sure your balance.
Order
You can use API about orders in exchange.
New order
Publish new order to exchange.
For example if you'd like buy 10 BTC as 30000 JPY/BTC, you need to specify following parameters.
rate: 30000, amount: 10, order_type: "buy", pair: "btc_jpy"
In case of buying order, if selling order which is lower than specify price is already exist, we settle in low price order. In case of to be short selling order, remain as unsettled order. It is also true for selling order.
About rate limit
New order to the exchange can be up to 5 requests per second.
If exceeded, it will return 429:too_many_requests error.
Limits are not per currency pair.
Limits may be changed depending on the system load status.
About order_type
There 4 types of order_type .
- "buy" Limit, Spot, Buy
- "sell" Limit, Spot, Sell
- "market_buy" Market, Spot, Buy
- "market_sell" Market, Spot, Sell
"buy"
Limit, Spot, Buy
- *rate Order rate. ex) 28000
- *amount Order amount. ex) 0.1
"sell"
Limit, Spot, Sell
- *rate Order rate. ex) 28000
- *amount Order amount. ex) 0.1
"market_buy"
Market, Spot, Buy
In MarketBuy you have to specify JPY not BTC.
- *market_buy_amount Market buy amount in JPY not BTC. ex) 10000
"market_sell"
Market, Spot, Sell
- *amount Order amount. ex) 0.1
HTTP REQUEST
POST /api/exchange/orders
PARAMETERS
- *pair Specify a currency pair to trade. btc_jpy, eth_jpy, etc_jpy, lsk_jpy, xrp_jpy, xem_jpy, bch_jpy, mona_jpy, iost_jpy, enj_jpy, chz_jpy, imx_jpy, shib_jpy, avax_jpy, plt_jpy, fnct_jpy, dai_jpy, wbtc_jpy, bril_jpy, bc_jpy, doge_jpy are now available.
- *order_type Specify order_type.
- rate Order rate. ex) 28000
- amount Order amount. ex) 0.1
- market_buy_amount Market buy amount in JPY not BTC. ex) 10000
- stop_loss_rate Stop Loss Rate (What is Stop Loss Order?)
- time_in_force Time In Force(optional)。"good_til_cancelled"(the order will last until it is completed or you cancel it. default action) or "post_only"(the order will exist as a maker order on the order book, but never match with orders that are already on the book)
{"success": true, "id": 12345, "rate": "30010.0", "amount": "1.3", "order_type": "sell", "time_in_force": "good_til_cancelled", "stop_loss_rate": null, "pair": "btc_jpy", "created_at": "2015-01-10T05:55:38.000Z"}
RESPONSE ITEMS
- id New order ID
- rate Order rate
- amount Order amount
- order_type Order type
- time_in_force Time In Force
- stop_loss_rate Stop Loss Rate
- pair Deal pair
- created_at Order date
Order details
You can get the details of the order.
About rate limit
Order details API calls can be requested to the exchange at most once per second.
If exceeded, it will return 429:too_many_requests error.
Limits are not per currency pair.
Limits may be changed depending on the system load status.
HTTP REQUEST
GET /api/exchange/orders/[id]
PARAMETERS
- *id Order ID(It's the same ID in New order.)
{ "success": true, "id": 12345, "pair": "btc_jpy", "status": "PARTIALLY_FILLED_EXPIRED", "order_type": "buy", "rate": "0.1", "stop_loss_rate": null, "maker_fee_rate": "0.001", "taker_fee_rate": "0.001", "amount": "1.0", "market_buy_amount": null, "executed_amount": "0", "executed_market_buy_amount": null, "expired_type": "self_trade_prevention", "prevented_match_id": 123, "expired_amount": "1.0", "expired_market_buy_amount": null, "time_in_force": "good_til_cancelled", "created_at": "2015-01-10T05:55:38.000Z" }
RESPONSE ITEMS
- id Order ID(It's the same ID in New order.)
- pair Deal pair
-
status
Order Status
- *NEW Order Received
- *WAITING_FOR_TRIGGER Before Trigger Conditions like Stoploss
- *PARTIALLY_FILLED Partially Filled
- *FILLED Fully Filled
- *CANCELED Canceled
- *EXPIRED Expired
- *PARTIALLY_FILLED_CANCELED Partially Filled and Canceled
- *PARTIALLY_FILLED_EXPIRED Partially Filled and Expired
- order_type Order type(It's the same order_type in New order.)
- rate Order rate
- stop_loss_rate Stop Loss Order's Rate
- maker_fee_rate Make fee rate
- taker_fee_rate Taker fee rate
- amount Order amount
- market_buy_amount Market buy amount
- executed_amount Executed amount
- executed_market_buy_amount Executed market buy amount
-
expired_type
Reasons for Expiration
- *self_trade_prevention Expired due to self-trade prevention
- *price_limit Expired due to price limit
- *post_only Expired because post-only order did not become a Maker
- *unfilled_market Expired because market order was not fully filled
- *itayose_market Market order expired while accepting limit orders
- prevented_match_id Prevented match ID
- expired_amount Expired amount
- expired_market_buy_amount Expired market buy amount
- time_in_force Time In Force
- created_at Order date
Unsettled order list
You can get a unsettled order list.
HTTP REQUEST
GET /api/exchange/orders/opens
{"success":true,"orders":[{"id":202835,"order_type":"buy","rate":26890,"pair":"btc_jpy","pending_amount":"0.5527", "pending_market_buy_amount": null,"stop_loss_rate": null, "created_at": "2015-01-10T05:55:38.000Z"},{"id":202836,"order_type":"sell","rate":26990,"pair":"btc_jpy","pending_amount":"0.77", "pending_market_buy_amount": null,"stop_loss_rate": null, "created_at": "2015-01-10T05:55:38.000Z"},{"id":38632107,"order_type":"buy","rate":null,"pair":"btc_jpy","pending_amount":null,"pending_market_buy_amount": "10000.0", "stop_loss_rate":"50000.0","created_at":"2016-02-23T12:14:50.000Z"}]}
RESPONSE ITEMS
- id Order ID(It's the same ID in New order.)
- rate Order rate ( Market order if null)
- pending_amount Unsettle order amount
- pending_market_buy_amount Unsettled order amount (only for spot market buy order)
- order_type order type("sell" or "buy")
- stop_loss_rate Stop Loss Order's Rate
- pair Deal pair
- created_at Order date
Cancel Order
New OrderOr, you can cancel by specifyingunsettle order list's ID.
HTTP REQUEST
DELETE /api/exchange/orders/[id]
PARAMETERS
- *id New order or Unsettle order list's ID
{"success": true, "id": 12345}
RESPONSE ITEMS
- id Canceled order ID
Order cancellation status
You can refer to the cancellation processing status of the order.
HTTP REQUEST
GET /api/exchange/orders/cancel_status?id=[id]
PARAMETERS
- *id New Order Or, you can cancel by specifyingunsettle order list's ID.
{"success": true, "id": 12345, "cancel": true, "created_at": "2020-07-29T17:09:33.000Z"}
RESPONSE ITEMS
- id Order ID
- cancel Canceled(true or false)※1
- created_at Ordered time
※1 Expired orders are also treated as canceled orders.
Transaction history
Display your transaction history
HTTP REQUEST
GET /api/exchange/orders/transactions
{"success":true,"transactions":[{"id":38,"order_id":49,"created_at":"2015-11-18T07:02:21.000Z","funds":{"btc":"0.1","jpy":"-4096.135"},"pair":"btc_jpy","rate":"40900.0","fee_currency":"JPY","fee":"6.135","liquidity":"T","side":"buy"},{"id":37,"order_id":48,"created_at":"2015-11-18T07:02:21.000Z","funds":{"btc":"-0.1","jpy":"4094.09"},"pair":"btc_jpy","rate":"40900.0","fee_currency":"JPY","fee":"-4.09","liquidity":"M","side":"sell"}]}
RESPONSE ITEMS
- id ID
- order_id Order ID
- created_at Ordered time
- funds Each fund balance's increase and decrease
- pair Pair
- rate Rate
- fee_currency Fee currency
- fee Fee amount
- liquidity "T" ( Taker ) or "M" ( Maker ) or "itayose" ( Itayose )
- side "sell" or "buy"
Transaction history (Pagination)
Display your transaction history
HTTP REQUEST
GET /api/exchange/orders/transactions_pagination
{"success":true,"pagination":{"limit":1,"order":"desc","starting_after":null,"ending_before":null},"data":[{"id":38,"order_id":49,"created_at":"2015-11-18T07:02:21.000Z","funds":{"btc":"0.1","jpy":"-4096.135"},"pair":"btc_jpy","rate":"40900.0","fee_currency":"JPY","fee":"6.135","liquidity":"T","side":"buy"},{"id":37,"order_id":48,"created_at":"2015-11-18T07:02:21.000Z","funds":{"btc":"-0.1","jpy":"4094.09"},"pair":"btc_jpy","rate":"40900.0","fee_currency":"JPY","fee":"-4.09","liquidity":"M","side":"sell"}]}
RESPONSE ITEMS
- id ID
- order_id Order ID
- created_at Ordered time
- funds Each fund balance's increase and decrease
- pair Pair
- rate Rate
- fee_currency Fee currency
- fee Fee amount
- liquidity "T" ( Taker ) or "M" ( Maker ) or "itayose" ( Itayose )
- side "sell" or "buy"
Account
You can get balance and various information.
Balance
Check your account balance.
It doesn't include jpy_reserved use unsettled orders injpy btc.
HTTP REQUEST
GET /api/accounts/balance
{"success": true, "jpy": "0.8401", "btc": "7.75052654", "jpy_reserved": "3000.0", "btc_reserved": "3.5002", "jpy_lending": "0", "btc_lending": "0.1", "jpy_lend_in_use": "0", "btc_lend_in_use": "0.3", "jpy_lent": "0", "btc_lent": "1.2", "jpy_debt": "0", "btc_debt": "0", "jpy_tsumitate": "10000.0", "btc_tsumitate": "0.4034"}
RESPONSE ITEMS
- jpy Balance for JPY
- btc Balance for BTC
- jpy_reserved Amount of JPY for unsettled buying order
- btc_reserved Amount of BTC for unsettled selling order
- jpy_lending Total amount of JPY in the lending account before applying for lending (Currently, JPY lending is not available)
- btc_lending Total amount of BTC in the lending account before applying for lending
- jpy_lend_in_use Total amount of JPY in the lending account for which a lending application has been made (Currently, JPY lending is not available)
- btc_lend_in_use Total amount of BTC in the lending account for which a lending application has been made
- jpy_lent Total amount of JPY lent in the lending account (Currently, JPY lending is not available)
- btc_lent Total amount of BTC lent in the lending account
- jpy_debt JPY borrowing amount
- btc_debt BTC borrowing amount
- jpy_tsumitate JPY reserving amount
- btc_tsumitate BTC reserving amount
Send Crypto Currency
Sending Crypto Currency to specified address
HTTP REQUEST
POST /api/send_money
PARAMETERS
- *remittee_list_id RemitteeList Id sending to
- *amount Amount
- *purpose_type Purpose Type
- purpose_details Purpose Details
{"remittee_list_id": 12345, "amount": "0.000001", "purpose_type": "payment_of_importing", "purpose_details": { "specific_items_of_goods": "食料品", "place_of_origin": "カナダ", "place_of_loading": "アメリカ" }}
PURPOSE TYPES
The following is a list of keys that can be specified for purpose_type. Depending on the value of purpose_type, it may be necessary to specify detailed information in object format in purpose_details.
- inheritance_or_donating Inheritance, gift of living expenses
-
payment_of_importing
Settlement of Import Payments
- Additional fields that need to be specified in purpose_details.
-
- *specific_items_of_goods Specific Items of Goods
- *place_of_origin Place of Origin
- *place_of_loading Place of Loading
-
payment_of_intermediary_trade
Settlement of Intermediary Trade Payments
- Additional fields that need to be specified in purpose_details.
-
- *specific_items_of_goods Specific Items of Goods
- *place_of_origin Place of Origin
- *place_of_loading Place of Loading
- *place_of_destination Place of Destination
- payment_of_domestic_transactions Settlement of payment for domestic transactions
- keep_own_account Keep at own account with other crypto asset exchange, other services
- keep_own_private_wallet Keep at own private wallet (e.g. MetaMask)
-
other
Other
- Additional fields that need to be specified in purpose_details.
-
- *extra_text Specific Purpose of the Transfer
{"success": true, "id": "276", "address": "1v6zFvyNPgdRvhUufkRoTtgyiw1xigncc", "amount": "1.5", "fee": "0.002"}
RESPONSE ITEMS
- id Send
- address Recipient's address
- amount Amount of sent
- fee fee
Sending History
BTC Sending history
HTTP REQUEST
GET /api/send_money
PARAMETERS
- *currency Currency(Only BTC)
{"success": true, "sends": [{ "id": 2, "amount": "0.05", "currency": "BTC", "fee": "0.0", "address": "13PhzoK8me3u5nHzzFD85qT9RqEWR9M4Ty", "created_at": "2015-06-13T08:25:20.000Z" }, { "id": 1, "amount": "0.05", "currency": "BTC", "fee": "0.0001", "address": "118ekvRwx6uc4241jkuQt5eYvLiuWdMW2", "created_at": "2015-06-13T08:21:18.000Z" }] }
RESPONSE ITEMS
- id Send
- amount Amount of bitcoins sent
- fee Fee
- currency Currency
- address Recipient's bitcoin address
- created_at Date you sent
Deposits History
BTC deposit history
HTTP REQUEST
GET /api/deposit_money
PARAMETERS
- *currency Currency(BTC now)
{"success": true, "deposits": [{ "id": 2, "amount": "0.05", "currency": "BTC", "address": "13PhzoK8me3u5nHzzFD85qT9RqEWR9M4Ty", "status": "confirmed", "confirmed_at": "2015-06-13T08:29:18.000Z", "created_at": "2015-06-13T08:22:18.000Z" }, { "id": 1, "amount": "0.01", "currency": "BTC", "address": "13PhzoK8me3u5nHzzFD85qT9RqEWR9M4Ty", "status": "received", "confirmed_at": "2015-06-13T08:21:18.000Z", "created_at": "2015-06-13T08:21:18.000Z" }] }
RESPONSE ITEMS
- id Received ID
- amount Amount of BTC received
- currency Currency
- address Bitcoin address you reveived BTC
- status Status
- confirmed_at Date Confirmed
- created_at Date when receiving process started
Account information
Display account information.
HTTP REQUEST
GET /api/accounts
{ "success": true, "id": 10000, "email": "test@gmail.com", "identity_status": "identity_pending", "bitcoin_address": "1v6zFvyNPgdRvhUufkRoTtgyiw1xigncc", "taker_fee": "0.15", "maker_fee": "0.0", "exchange_fees": { "btc_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "eth_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "etc_jpy": { "maker_fee_rate": "0.05", "taker_fee_rate": "0.1" }, "lsk_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "xrp_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "xem_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "bch_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "mona_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "iost_jpy": { "maker_fee_rate": "0.05", "taker_fee_rate": "0.1" }, "enj_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "chz_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "imx_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "shib_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "avax_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "plt_jpy": { "maker_fee_rate": "0.05", "taker_fee_rate": "0.1" }, "fnct_jpy": { "maker_fee_rate": "0.05", "taker_fee_rate": "0.1" }, "dai_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "wbtc_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" }, "bril_jpy": { "maker_fee_rate": "0.05", "taker_fee_rate": "0.1" }, "bc_jpy": { "maker_fee_rate": "0.05", "taker_fee_rate": "0.1" }, "doge_jpy": { "maker_fee_rate": "0.0", "taker_fee_rate": "0.0" } } }
RESPONSE ITEMS
- id Account ID. It's the same as the ID you specify when you deposit JPY.
- email Registered e-mail
- identity_status Your identity status.
- bitcoin_address Your bitcoin address for deposit
- taker_fee It displays the fee rate (%) in the case of performing the order as Taker.(BTC_JPY)
- maker_fee It displays the fee rate (%) in the case of performing the order as Maker.(BTC_JPY)
- exchange_fees It displays the fee for each order book.
Withdraw JPY
You can withdraw JPY through this API.
Bank account list
Display list of bank account you registered (withdrawal).
HTTP REQUEST
GET /api/bank_accounts
{"success":true,"data":[{"id":243,"bank_name":"みずほ","branch_name":"東京営業部","bank_account_type":"futsu","number":"0123456","name":"タナカ タロウ"}]}
RESPONSE ITEMS
- id ID
- bank_name Bank name
- branch_name Branch name
- bank_account_type Type of bank account
- number Bank account number
- name Bank account name
Register bank account
Register bank account for withdrawal.
HTTP REQUEST
POST /api/bank_accounts
PARAMETERS
- *bank_name Bank name
- *branch_name Branch name
- *bank_account_type Type of bank account ( "futsu" or "toza" )
- *number Bank account number (ex) "0123456"
- *name Bank account name
{"success":true,"data":{"id":641,"bank_name":"熊本","branch_name":"田中","bank_account_type":"futsu","number":"0123456","name":"hoge"}}
RESPONSE ITEMS
- id ID
- bank_name Bank name
- branch_name Branch name
- bank_account_type Type of bank account ( "futsu" or "toza" )
- number Bank account number (ex) "0123456"
- name Bank account name
Remove bank account
Will remove your bank account.
HTTP REQUEST
DELETE /api/bank_accounts/[id]
PARAMETERS
- *id Bank account list iD
{"success":true}
Withdraw history
Display Japanese YEN withdrawal request history.
HTTP REQUEST
GET /api/withdraws
{"success":true,"pagination":{"limit":25,"order":"desc","starting_after":null,"ending_before":null},"data":[{"id":398,"status":"finished","amount":"242742.0","currency":"JPY","created_at":"2014-12-04T15:00:00.000Z","bank_account_id":243,"fee":"400.0", "is_fast": true}]}
RESPONSE ITEMS
- id ID
- status Withdraw status ( pending, processing, finished, canceled )
- amount Amount
- currency Currency
- created_at Date you created
- bank_account_id Bank account ID
- fee Fee
- is_fast Fast withdraw option. Currently stopped.
Create withdraw
Request Japanese Yen withdrawal
HTTP REQUEST
POST /api/withdraws
PARAMETERS
- *bank_account_id Bank account ID
- *amount Amount
- *currency Currency ( only "JPY" )
{"success":true,"data":{"id":1043,"status":"pending","amount":"10000.0","currency":"JPY","created_at":"2015-08-31T11:32:45.213Z","bank_account_id":243,"fee":"300.0"}}
RESPONSE ITEMS
- id ID
- status Withdraw status ( pending, processing, finished, canceled )
- amount Amount
- currency Currency ( only "JPY" )
- created_at Date you created
- bank_account_id Bank account ID
- fee Fee
Cancel withdrawal
Cancel withdrawal request.
You can only cancel a withdrawal request that has the pending status.
HTTP REQUEST
DELETE /api/withdraws/[id]
PARAMETERS
- *id Withdraw ID
{"success":true}
WebSocket API (beta)
The WebSocket API enables real-time transaction that is hard to implement with HTTP. With WebSocket API you can fetch Coincheck orderbook information and transaction history seamlessly.
Since the WebSocket API is still in beta, the specification may change, or operation may be unstable. Also, btc_jpy, eth_jpy, etc_jpy, lsk_jpy, xrp_jpy, xem_jpy, bch_jpy, mona_jpy, iost_jpy, enj_jpy, chz_jpy, imx_jpy, shib_jpy, avax_jpy, plt_jpy, fnct_jpy, dai_jpy, wbtc_jpy, bril_jpy, bc_jpy, doge_jpy are available as currency pairs. (As of September 30, 2020)
Overview
WebSocket Connection
wss://ws-api.coincheck.com/
Subscribe
To begin receiving messages, you must first send a subscribe message to the server indicating which product messages to receive. This message must be JSON format.
Example
//JavaScript
//Run in Chrome
socket = new WebSocket("wss://ws-api.coincheck.com/")
socket.send(JSON.stringify({type: "subscribe", channel: "btc_jpy-orderbook"}))
socket.send(JSON.stringify({type: "subscribe", channel: "btc_jpy-trades"}))
Public Channels
Public channel is a channel that does not require authentication.
Public trades
The Public Trades Channel pushes information on recent transactions every 0.1 seconds.
REQUEST ITEMS
{"type":"subscribe","channel":"[pair]-trades"}
RESPONSE ITEMS
["transaction timestamp (unix time)", "transaction ID","pair","transaction rate","transaction amount","order side","ID of the Taker","ID of the Maker"]
※ Only the takers' order information is pushed through the stream.
REQUEST EXAMPLE
{"type":"subscribe","channel":"btc_jpy-trades"}
RESPONSE EXAMPLES
※ The transaction information will be pushed as a 2-dimensional array.
[["1663318663","2357062","btc_jpy","2820896.0","5.0","sell","1193401","2078767"],["1663318892","2357068","btc_jpy","2820357.0","0.7828","buy","4456513","8046665"]]
Order Book
Send the difference of order book information at regular intervals.
REQUEST ITEMS
{"type":"subscribe", "channel":"[pair]-orderbook"}
RESPONSE ITEMS
["[pair]",{"bids":[["order rate","order amount"],["order rate","order amount"]],"asks":[["order rate","order amount"],["order rate","order amount"]], "last_update_at": "timestamp (unix time)"}]
※ “bids“ refer to buy orders, and “asks“ refer to sell orders.
REQUEST EXAMPLE
{"type":"subscribe", "channel":"btc_jpy-orderbook"}
RESPONSE EXAMPLES
["btc_jpy",{"bids":[["148634.0","0.12"],["148633.0","0.0574"]],"asks":[["148834.0","0.0812"],["148833.0","1.0581"]],"last_update_at":"1659321701"}]